๐ You can now mutate meta values in Gato GraphQL
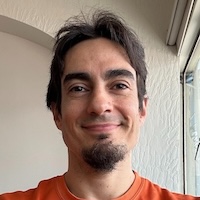
v11.3
of Gato GraphQL was released today, with support for an important feature: Meta mutations!
You can now add, update, and delete meta values, for custom posts, tags, categories, comments, and users.
Below are examples of queries mutating meta.
Adding meta
You can add meta entries to custom posts, tags, categories, comments, and users.
This query adds a meta entry to post with ID 4
:
mutation {
addCustomPostMeta(input: {
id: 4
key: "some_key"
value: "Some value"
}) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
customPost {
id
metaValue(key: "some_key")
}
}
}
This query adds the same meta key with different values to different posts, in bulk:
mutation {
addCustomPostMetas(inputs: [
{
id: 4
key: "some_key"
value: "Some value"
},
{
id: 5
key: "some_key"
value: "Some other value"
},
{
id: 6
key: "some_key"
value: "Yet another value"
}
]) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
customPost {
id
metaValue(key: "some_key")
}
}
}
Updating meta
Update a category meta entry:
mutation {
updateCategoryMeta(input: {
id: 20
key: "_source"
value: "Updated source value"
}) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
category {
__typename
id
metaValue(key: "_source")
}
}
}
This query uses nested mutations to update a meta value in a post:
mutation {
post(by: {id: 1}) {
updateMeta(input: {
key: "some_key"
value: "Updated description"
}) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
post {
id
metaValue(key: "single_meta_key")
}
}
}
}
Deleting meta
Delete a meta entry from a post:
mutation {
deletePostMeta(input: {
id: 5
key: "some_key"
}) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
post {
id
metaValue(key: "some_key")
}
}
}
Delete the same meta entry from multiple posts, in bulk:
mutation {
deletePostMetas(inputs: [
{
id: 5
key: "some_key"
},
{
id: 6
key: "some_key"
}
]) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
post {
id
metaValue(key: "some_key")
}
}
}
Setting multiple meta entries at once
You can set multiple meta entries at once by passing a JSON to the different set{Entity}Meta
mutations:
mutation {
setCustomPostMeta(input: {
id: 4
entries: {
single_meta_key: [
"This is a single entry",
],
object_meta_key: [
{
key: "This is a key",
value: "This is a value",
},
],
array_meta_key: [
"This is a string",
"This is another string",
],
object_array_meta_key: [
[
{
key: "This is a key 1",
value: "This is a value 1",
},
{
key: "This is a key 2",
value: "This is a value 2",
},
]
],
}
}) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
customPost {
id
meta(keys: ["single_meta_key", "object_meta_key", "array_meta_key", "object_array_meta_key"])
}
}
}
Setting meta entries when creating/updating an entity
You can define meta entries directly when creating or updating a custom post, tag, category, or comment, via param meta
.
This query sets meta when adding a comment:
mutation {
addCommentToCustomPost(input: {
customPostID: 1130
commentAs: { html: "New comment" }
meta: {
some_meta_key: [
"This is a single entry",
],
another_meta_key: [
"This is an array entry 1",
"This is an array entry 2",
],
}
}) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
comment {
id
meta(keys: ["some_meta_key", "another_meta_key"])
}
}
}
This query injects the meta in nested mutation Post.update
:
mutation {
post(by: {id: 1}) {
update(input: {
meta: {
single_meta_key: [
"This is an updated value",
]
}
}) {
status
errors {
__typename
...on ErrorPayload {
message
}
}
post {
id
metaValue(key: "single_meta_key")
}
}
}
}